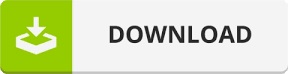
The Deque-specific methods were added with the introduction of Deque in Java 6. It has existed in the JDK since version 1.2, significantly longer than the Deque interface it implements. dequeue () removes the last element of the queue. There are 5 primary operations in Queue: enqueue () adds element x to the front of the queue. You can see my method below this picture. The class implements a classic doubly linked list. We can implement Queue for not only Integers but also Strings, Float, or Characters. However I am struggling with the concept of adding new nodes as I cant manage to link the pre-existing nodes together using FIFO. working with a Queue as a LinkedList adding elements to the back of the list and removing them from the front. ("Expected size 0, Actual size: " + myQueue.size()) Stack and queues using doubly linked list in java. Although there are more efficient ways to implement priority queues, especially for large datasets, this method serves as a foundational understanding of how priority-based data structures work. Linked Lists in C - Linked List Data Structure - GeeksforGeeks. ("Expected removed value 3, Actual removed value: " + i) Implementing a priority queue using a linked list in Java provides an intuitive way to manage priority data.
#Java queue linked list code#
And please note: your code shows a potential solution. We will implement Queue using linked list. A small warning: if you dont use poll or peek and only iterator () (+.remove ()) it will leak memory.
#Java queue linked list free#
you change the underlying data structure to something that allows 'random' remove actions. The Queue implemented using linked list can organize as many data values as we want. ConcurrentLinkedQueue is a superb lock free queue and does what a concurrent single linked list can do.
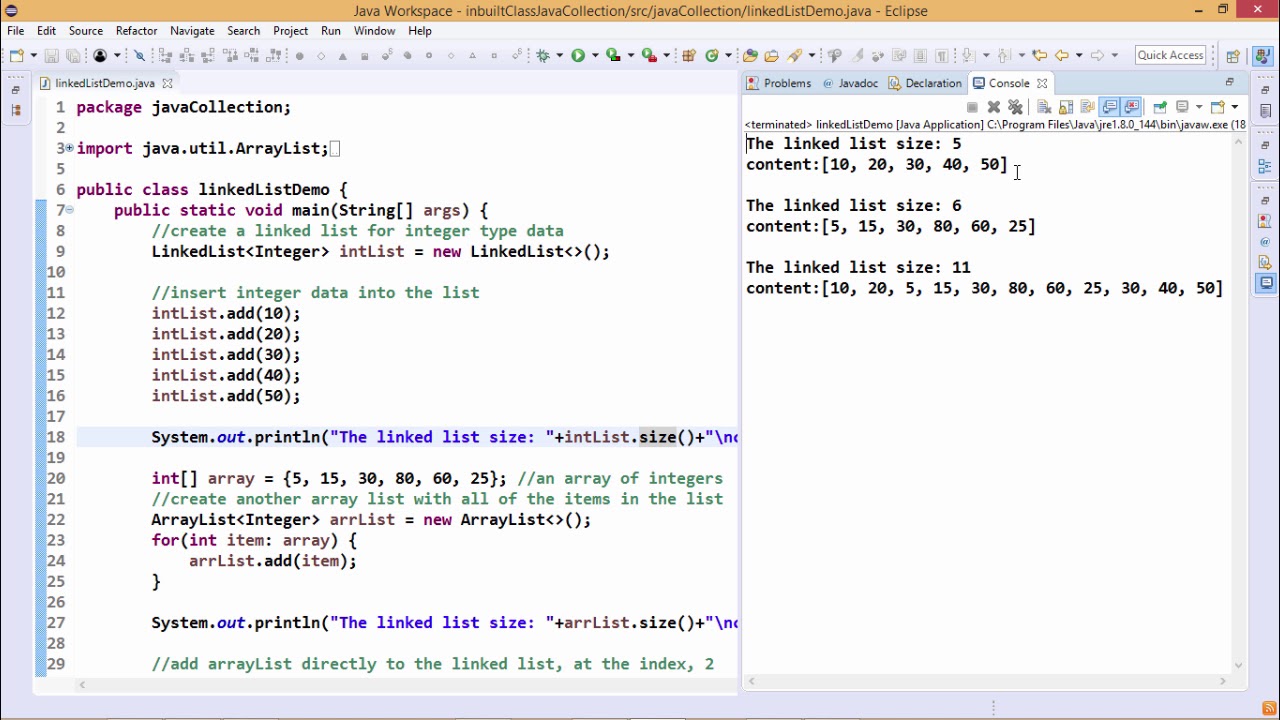
("Expected removed value 2, Actual removed value: " + i) you either have to 'pop' all entries of the queue up to the one to remove, to then add them back. ("Expected removed value 1, Actual removed value: " + i) Creating linked lists and queues in Java are rather. linked lists are indexed starting with zero. so we can easily add or remove by using add and. In Java or C we have a inbuilt class libary called linkedlist. A node of a doubly linked list has anext and a prev link. Queue is a first in first out data structure. To implement a deque, we use adoubly linked list.
("Expected size 2, Actual size: " + myQueue.size()) In this lab, you will switch to implementing a singly-linked queue using a linked-list to store data. Consider these statements: LinkedList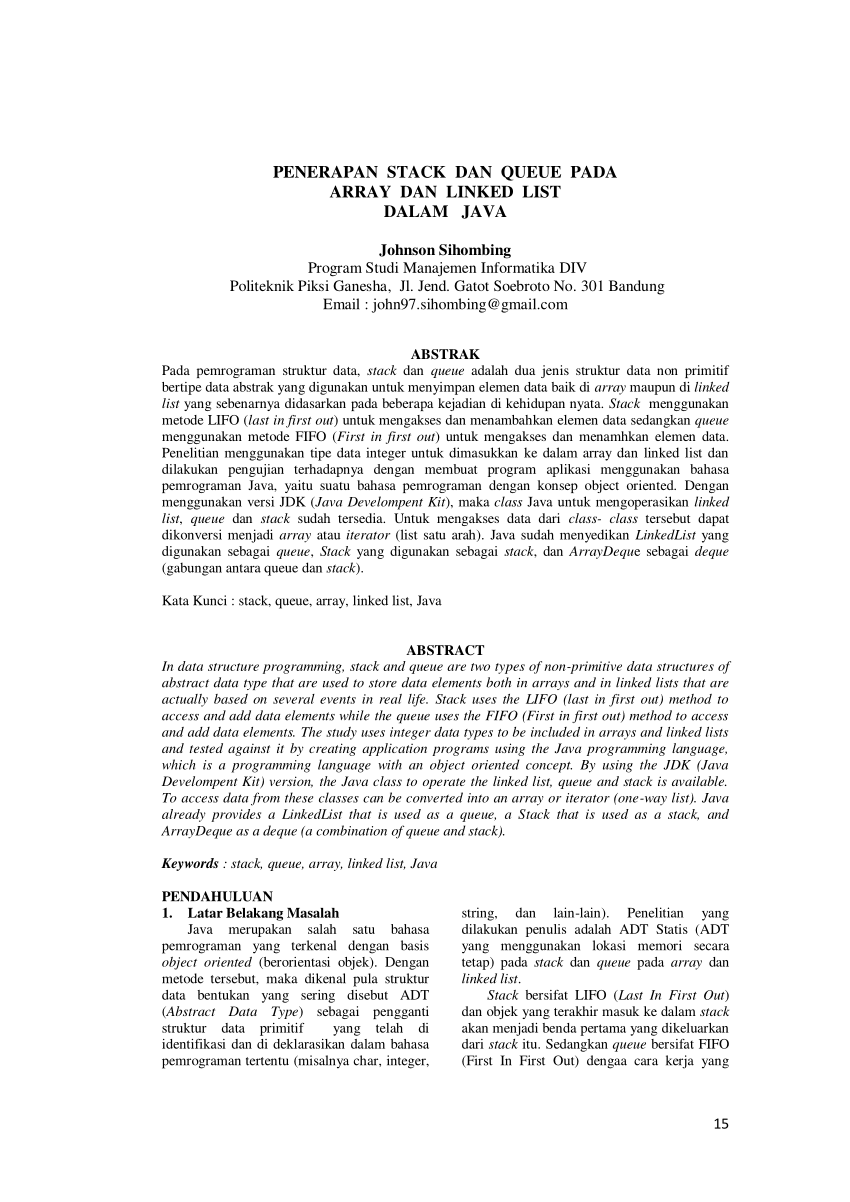
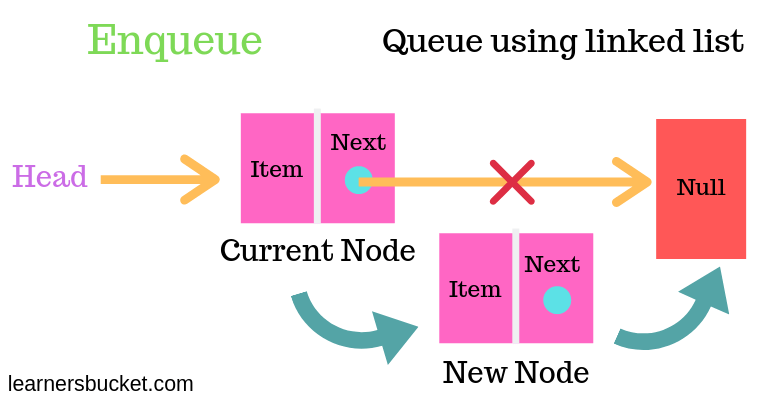

("Expected size 3, Actual size: " + myQueue.size()) There is the method add for adding an object, and there is the method remove for removing an object off the queue, as well as some other utility methods such as peek, size, isEmpty. remove (): Removes and returns the element at the front of the queue. offer (element): Adds an element to the rear of the queue. If the queue is full, it throws an exception. In the following queue implementation, it uses linked list. Here are some of the most commonly used methods: add (element): Adds an element to the rear of the queue. It’s like people queuing up for getting in to a restaurant. Queue is a first in first out, last in last out data structure.
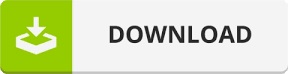